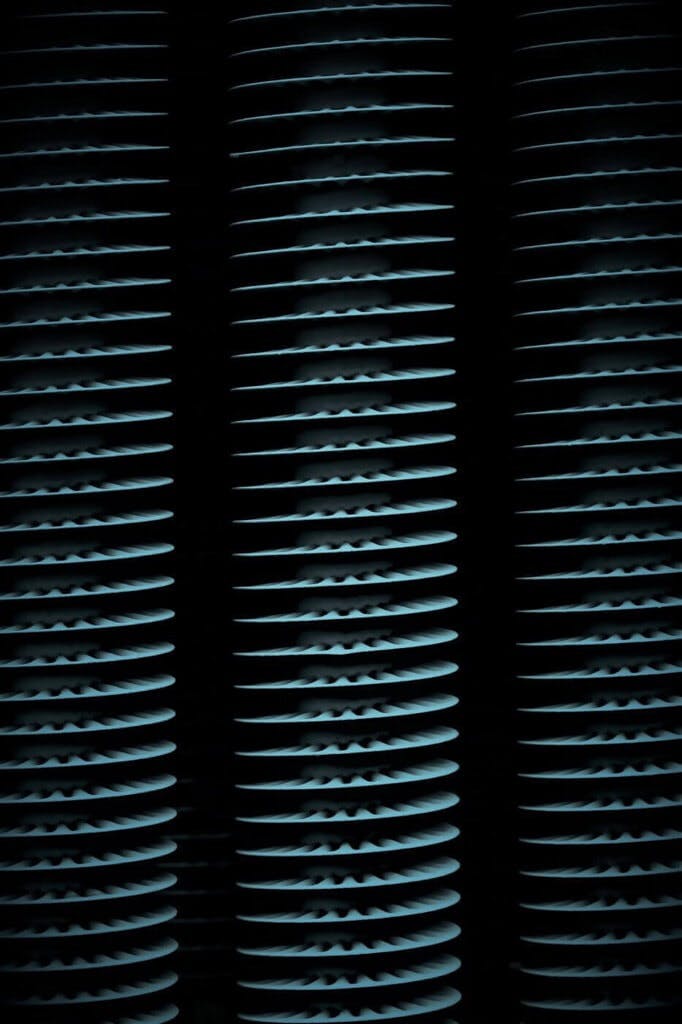
Your first Kubernetes Pod and ReplicaSet (LABS)
You've created your Kubernetes cluster and now it's time to put it to use. In this second lab you'll create your first Kubernetes Pod and your first Kubernetes ReplicaSet
Running your first Kubernetes Pods
apiVersion: v1
kind: Pod
metadata:
name: hello-pod
labels:
app.kubernetes.io/name: hello
spec:
containers:
- name: hello-container
image: busybox
command: ['sh', '-c', 'echo Hello from my container! && sleep 3600']
pod.yaml
and use the following command to create the Pod:kubectl apply -f pod.yaml
pod/hello-pod created
kubectl get pods
to list all Pods running the default
namespace of the cluster.$ kubectl get pods
NAME READY STATUS RESTARTS AGE
hello-pod 1/1 Running 0 7s
logs
command to see the output from the container running inside the Pod:$ kubectl logs hello-podHello from my container!
Note
When running multiple containers running inside the same Pod, you can use the-c
flag to specify the container name whose logs you want to get. For example:kubectl logs hello-pod -c hello-container
kubectl delete pod hello-pod
, the Pod will be gone forever because nothing would automatically restart it.kubectl get pods
again, you will notice the Pod is gone:$ kubectl get pods
No resources found in default namespace.
Keeping the Pods running with ReplicaSets
replicas
field in the resource definition.apiVersion: apps/v1
kind: ReplicaSet
metadata:
name: hello
labels:
app.kubernetes.io/name: hello
spec:
replicas: 5
selector:
matchLabels:
app.kubernetes.io/name: hello
template:
metadata:
labels:
app.kubernetes.io/name: hello
spec:
containers:
- name: hello-container
image: busybox
command: ['sh', '-c', 'echo Hello from my container! && sleep 3600']
replicaset.yaml
and use the following command to create the ReplicaSet:kubectl apply -f replicaset.yaml
$ kubectl get replicaset
NAME DESIRED CURRENT READY AGE
hello 5 5 5 30s
$ kubectl get po
NAME READY STATUS RESTARTS AGE
hello-dwx89 1/1 Running 0 31s
hello-fchvr 1/1 Running 0 31s
hello-fl6hd 1/1 Running 0 31s
hello-n667q 1/1 Running 0 31s
hello-rftkf 1/1 Running 0 31s
kubectl get po -l=app.kubernetes.io/name=hello
, we will get all Pods that have app.kubernetes.io/name: hello
label set. The output includes the five Pods we created.ownerReferences
field. We can use the -o yaml
flag to get the YAML representation of any object in Kubernetes. Once we get the YAML, we will search for the ownerReferences
string:$ kubectl get po hello-dwx89 -o yaml | grep -A5 ownerReferences
---
ownerReferences:
- apiVersion: apps/v1
blockOwnerDeletion: true
controller: true
kind: ReplicaSet
name: hello
ownerReferences
, Kubernetes sets the name
to hello
, and the kind
to ReplicaSet
. The name corresponds to the ReplicaSet that owns the Pod.po
in the command to refer to Pods? Some Kubernetes resources have short names you can use in place of the 'full names'. You can use po
when you mean pods
or deploy
when you mean deployment
. To get the full list of supported short names, you can run kubectl api-resources
.hello-pod
, because that's what we specified in the YAML. Using the ReplicaSet, Kubernetes names the Pods using the combination of the ReplicaSet name (hello
) and a semi-random string such as dwx89
, fchrv
and so on.delete
keyword followed by the resource (e.g. pod
) and the resource name hello-dwx89
(note that your Pod will have a different name):$ kubectl delete po hello-dwx89
pod "hello-dwx89" deleted
kubectl get pods
again. Did you notice something? There are still five Pods running.$ kubectl get po
NAME READY STATUS RESTARTS AGE
hello-fchvr 1/1 Running 0 14m
hello-fl6hd 1/1 Running 0 14m
hello-n667q 1/1 Running 0 14m
hello-rftkf 1/1 Running 0 14m
hello-vctkh 1/1 Running 0 48s
AGE
column, you will notice four Pods created 14 minutes ago and one created more recently. ReplicaSet created this new Pod. When we deleted one Pod, the number of actual replicas decreased from five to four. The replica set controller detected that and created a new Pod to match the replicas' desired number (5).app.kubernetes.io/name: hello
).apiVersion: v1
kind: Pod
metadata:
name: stray-pod
labels:
app.kubernetes.io/name: hello
spec:
containers:
- name: stray-pod-container
image: busybox
command: ['sh', '-c', 'echo Hello from stray pod! && sleep 3600']
stray-pod.yaml
file, then create the Pod by running:$ kubectl apply -f stray-pod.yaml
pod/stray-pod created
kubectl get pods
you will notice that the stray-pod
has disappeared. What happened?stray-pod
with the label app.kubernetes.io/name=hello
that matches the selector label on the ReplicaSet, the ReplicaSet took that new Pod for its own. Remember, the manually created Pod didn't have the owner. With this new Pod under ReplicaSets' management, the number of replicas was six and not five, as stated in the ReplicaSet. Therefore, the ReplicaSet did what it's supposed to do; it deleted the new Pod to maintain the desired state of five replicas.Zero-downtime deployments
busybox
to busybox:1.31.1
. We could use kubectl edit rs hello
to open the replica set YAML in the editor, then update the image value.$ kubectl describe po hello-fchvr | grep image
Normal Pulling 14m kubelet, docker-desktop Pulling image "busybox"
Normal Pulled 13m kubelet, docker-desktop Successfully pulled image "busybox"
busybox
image, but there's no sign of the busybox:1.31.1
anywhere. Let's see what happens if we delete this same Pod:$ kubectl delete po hello-fchvr
pod "hello-fchvr" deleted
hello-q8fnl
in this case) to match the desired replica count from the previous test we did. If we run describe
against the new Pod that came up, you will notice how the image has changed:$ kubectl describe po hello-q8fnl | grep image
Normal Pulling 74s kubelet, docker-desktop Pulling image "busybox:1.31"
Normal Pulled 73s kubelet, docker-desktop Successfully pulled image "busybox:1.31"
busybox
). The ReplicaSet would start new Pods, and this time, the Pods would use the new image busybox:1.31.1
.Cleanup
kubectl delete rs hello
. rs
is the short name for replicaset
. If you list the Pods (kubectl get po
) right after you issued the delete command, you will see the Pods getting terminated:NAME READY STATUS RESTARTS AGE
hello-fchvr 1/1 Terminating 0 18m
hello-fl6hd 1/1 Terminating 0 18m
hello-n667q 1/1 Terminating 0 18m
hello-rftkf 1/1 Terminating 0 18m
hello-vctkh 1/1 Terminating 0 7m39s